Overview
This project portfolio serves to document my role and contributions to the ClinicIO project, and to showcase my software engineering work to potential employers, academic programs, and other interested parties.
ClinicIO was developed by a team of five undergraduate students, as a project for a software engineering module. As a desktop application, ClinicIO aims to alleviate the administrative workload that doctors and receptionist face in their clinics every day.
ClinicIO provides users with the ability to manage patient records, schedule appointments, maintain a patient queue, organize a medicine inventory, and analyse clinic data.
Due to project constraints, ClinicIO was developed to be operated mainly by the command line, with a visual graphical interface for its users. Users will need to type commands to access the features of the application, instead of the usual mouse-clicking.
My team consisted of a team leader, an assistant team leader, and three other developers, each with their own responsibilities.
As a developer responsible for code integration, I was in charge of versioning the code, maintaining the code repository, and integrating various parts of the software to create a working product. Additionally, I was also in charge of the Storage component of ClinicIO, and designed the Medicine Inventory feature of the application.
Summary of contributions
This section serves to outline the contributions I have made to the development of ClinicIO. This include major and minor enhancements, code contributed, and other contributions made to my team and project. |
Major Enhancement 1: added the ability to add and delete medicines in the clinic’s Medicine Inventory
-
What it does: allows the user to add and delete medicines in the medicine inventory.
-
Justification: This feature improves the product significantly because a target user will need an efficient way to update the list of medicines in the clinic’s medicine inventory
-
Highlights: This enhancement adds new commands to the existing software.
Major Enhancement 2: added the ability to find and view medicines in the clinic’s Medicine Inventory
-
What it does: allows the user to find and view medicines in the medicine inventory.
-
Justification: A target user will need an efficient way to find and view the details of specific medicines in the clinic’s medicine inventory
-
Highlights: This enhancement adds new commands to the existing software.
Major Enhancement 3: added the ability to update medicine quantities in the clinic’s Medicine Inventory
-
What it does: allows the user to increase and decrease the quantities of medicines in the clinic’s medicine inventory.
-
Justification: A target user will need an efficient way to update the quantities of medicines when either prescribing medicines to a patient, or restocking medicines for future prescriptions.
-
Highlights: This enhancement adds new commands to the existing software.
Code contributed:
The link below displays all the code I have contributed to the ClinicIO project.
Other contributions:
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide.
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Medicine Inventory Commands
Adding a medicine: addmed
Adds a medicine to the medicine inventory
You must log-in as a receptionist in order to use this feature. |
Format: addmed mn/MEDICINE_NAME mt/MEDICINE_TYPE ed/EFFECTIVE_DOSAGE ld/LETHAL_DOSAGE mp/PRICE mq/QUANTITY
The lethal dosage of a Medicine should be more than its effective dosage.
|
Example:
-
addmed mn/fedac mt/tablet ed/2 ld/8 mp/0.09 mq/1500
Usage: (refer to screenshots below)
-
Enter the command in the correct format to add a medicine to the medicine inventory.
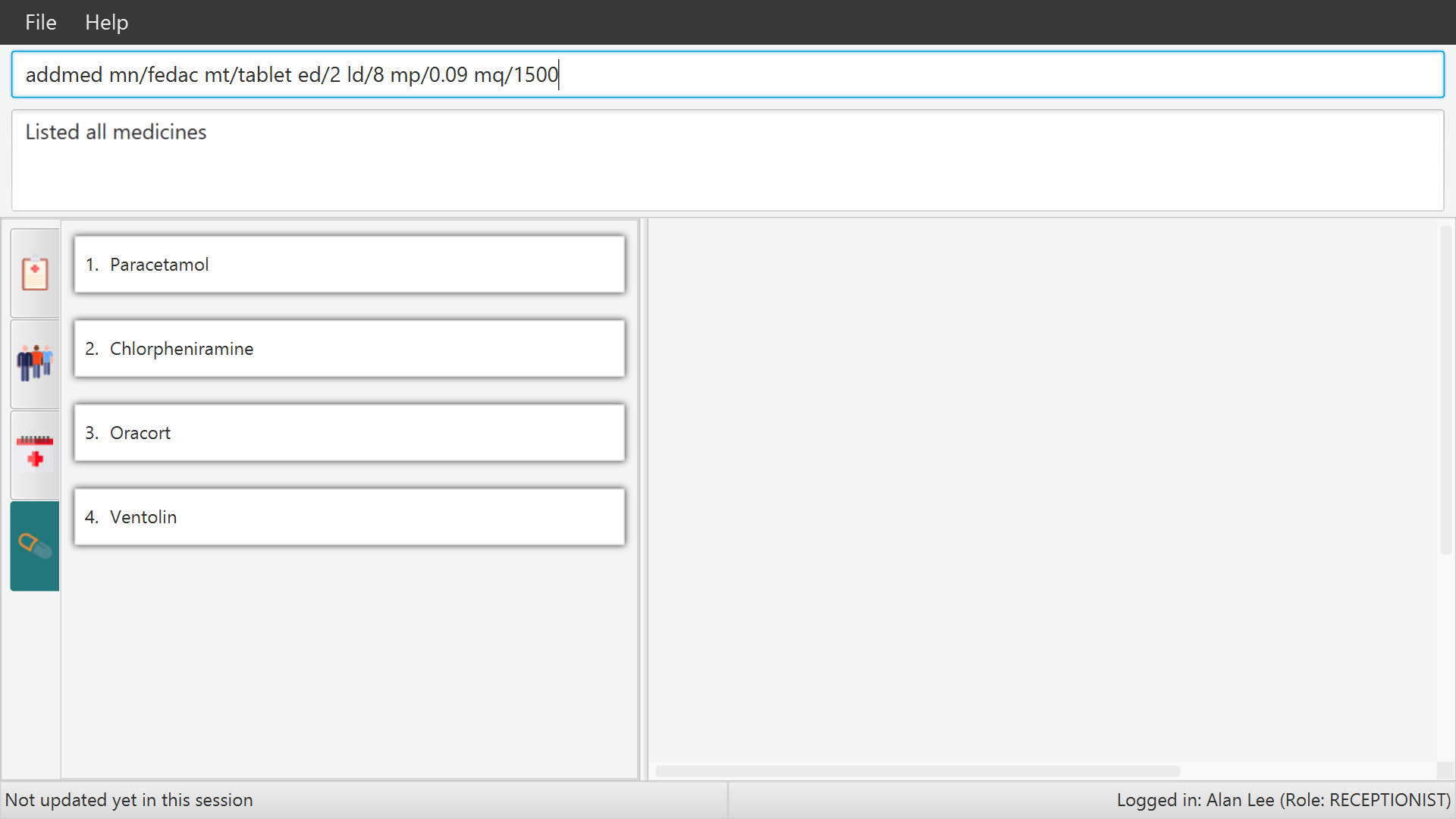
-
After successfully entering the command, you will see the newly added medicine in the displayed list of medicines.

Finding a medicine by name: findmed
Find a medicine in the medicine inventory
Format: findmed MEDICINE_NAME
Example:
-
findmed ibuprofen
Returns the details ofIbuprofen
Listing all medicines: listmed
Displays a list of all the medicines in the medicine inventory.
Format: listmed
Example:
-
listmed
Usage: (refer to screenshot below)
-
After successfully entering the command, you will see a list of medicines in the medicine inventory.
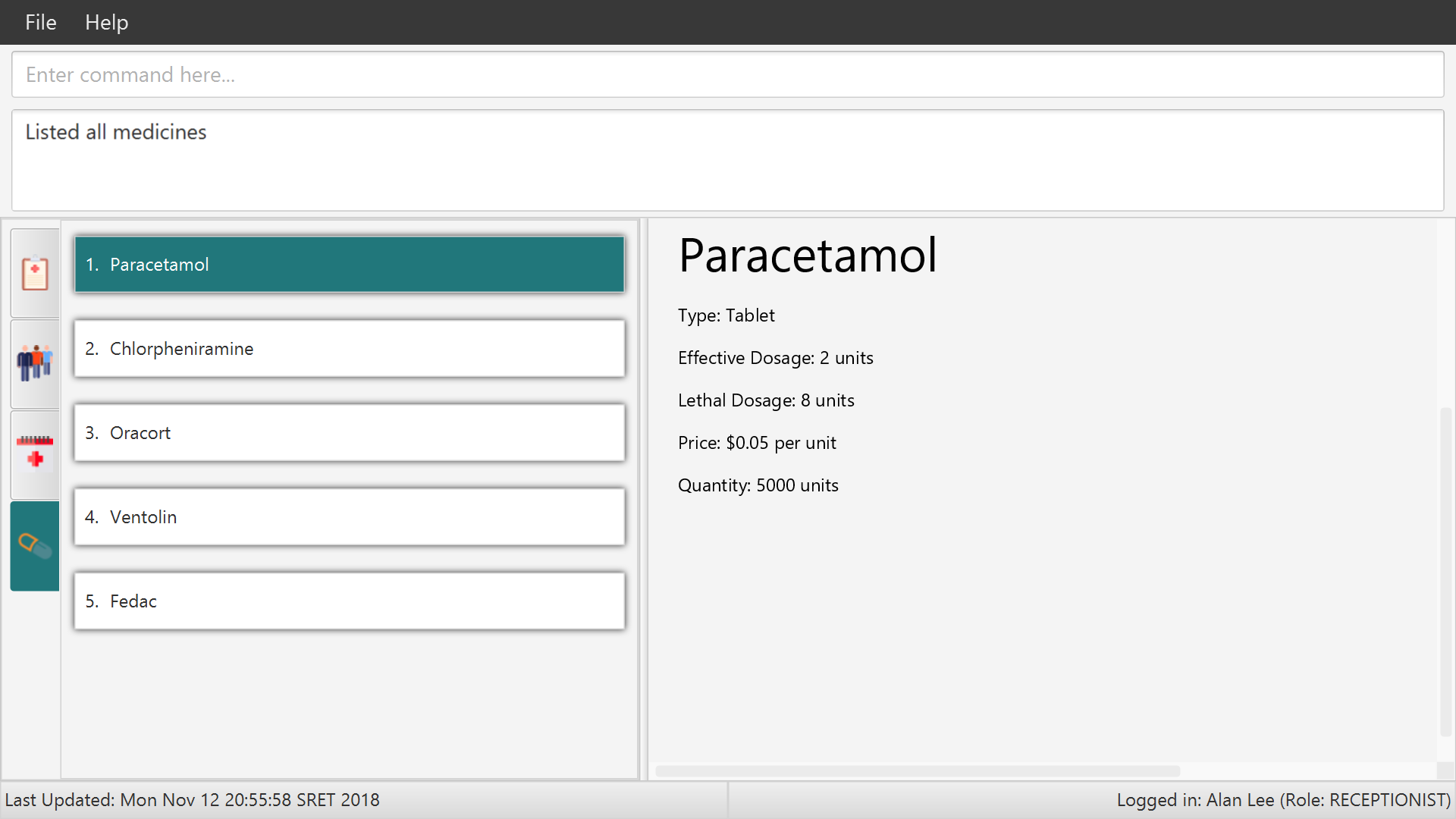
Removing a medicine by index : deletemed
Removes the specified medicine from the medicine inventory.
You must log-in as a receptionist in order to use this feature. |
Format: deletemed INDEX
Example:
-
listmed
Displays list of medicines in the medicine inventory. -
deletemed 1
Removes the first entry in the list of medicines.
Usage: (refer to screenshots below)
-
Enter the command in the correct format to remove a medicine from the medicine inventory.
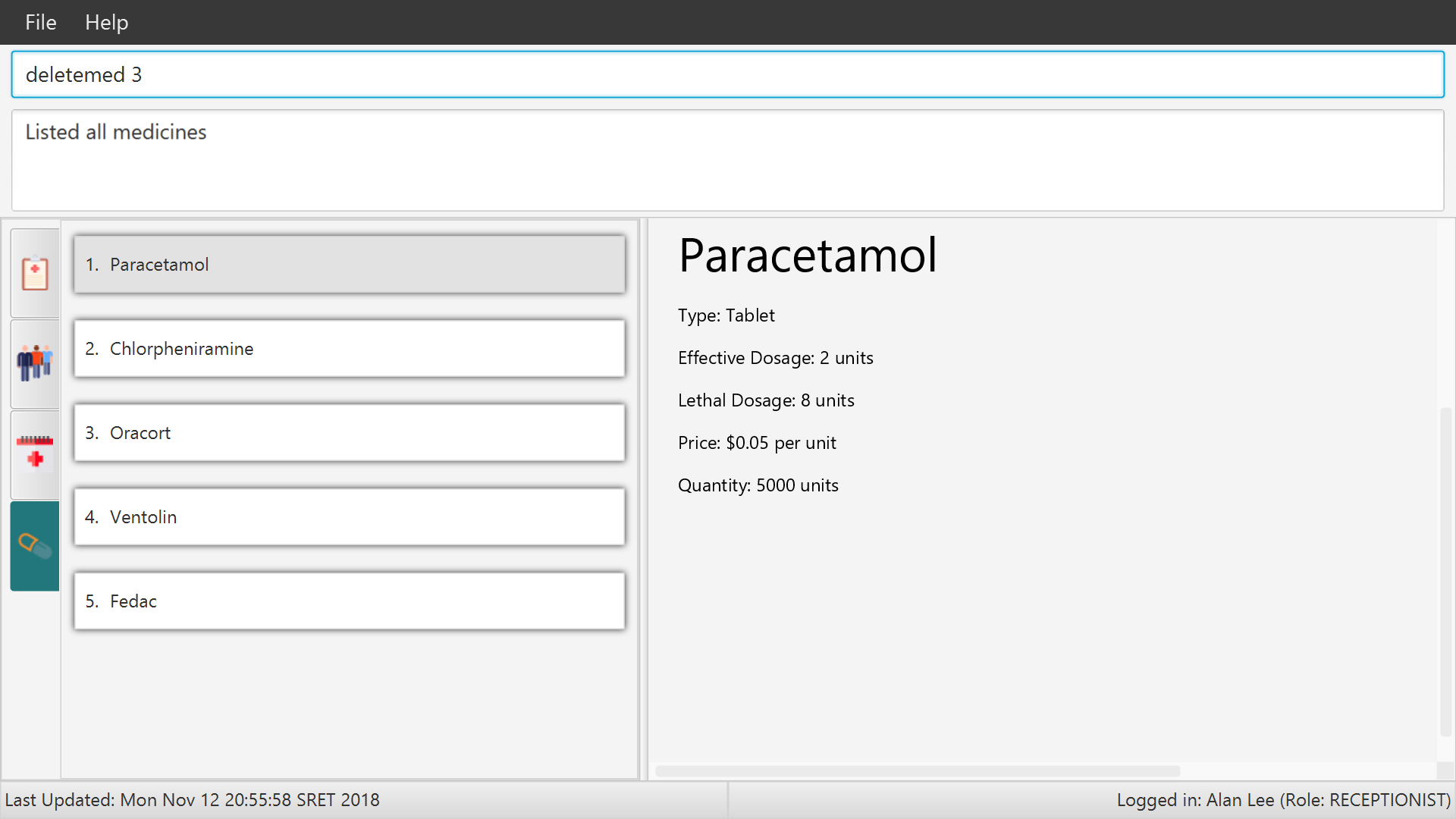
-
After successfully entering the command, you will see the updated displayed list of medicines without the removed medicine.
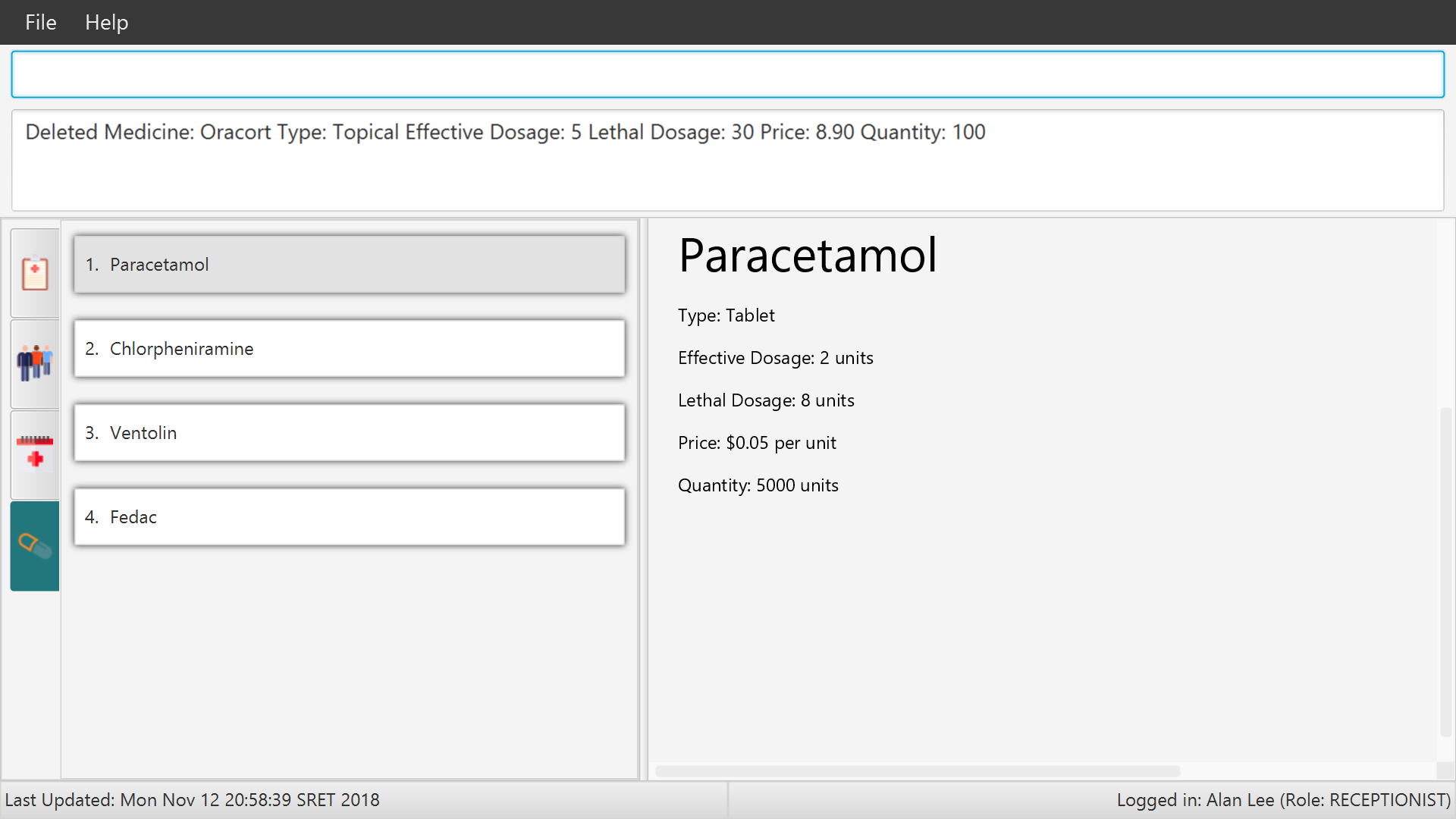
Selecting a medicine by index : selectmed
Selects the specified medicine in the displayed list of medicines.
Format: selectmed INDEX
Example:
-
listmed
Displays a list of medicines in the medicine inventory. -
selectmed 3
Selects the third entry in the list of medicines.
Increasing quantity of a medicine : increasemed
[coming in v2.0]
Increases the quantity of the specified medicine in the displayed list of medicines.
Format: increasemed INDEX mq/QUANTITY
Example:
-
listmed
Displays a list of medicines in the medicine inventory. -
increasemed 3 mq/500
Increase the quantity of the third entry in the list of medicines by 500 units.
Decreasing quantity of a medicine : decreasemed
[coming in v2.0]
Decreases the quantity of the specified medicine in the displayed list of medicines.
Format: decreasemed INDEX mq/QUANTITY
Example:
-
listmed
Displays a list of medicines in the medicine inventory. -
decreasemed 2 mq/10
Decrease the quantity of the second entry in the list of medicines by 10 units.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
MedicineInventory
Current Implementation
The MedicineInventory
extends ClinicIO
with a medicine inventory. The MedicineInventory
provides the user with an
organised system to manage the medicines in the clinic.
It implements the following operations:
-
MedicineInventory#hasMedicine(MedicineName medicineName)
— Checks if theMedicineInventory
contains theMedicine
with namemedicineName
. -
MedicineInventory#addMedicine(MedicineName medicineName, Medicine newMedicine)
— AddsnewMedicine
to theMedicineInventory
with itsmedicineName
. -
MedicineInventory#updateMedicineQuantity(MedicineName medicineName, MedicineQuantity newQuantity)
— Updates theMedicineQuantity
of theMedicine
with namemedicineName
with thenewQuantity
in theMedicineInventory
. -
MedicineInventory#deleteMedicine(MedicineName medicineName)
— Deletes theMedicine
with namemedicineName
from theMedicineInventory
.
These operations are exposed in the Model
interface as Model#hasMedicine(MedicineName medicine)
,
Model#addMedicine(MedicineName medicineName, Medicine medicine)
, Model#updateMedicine(MedicineName target,
MedicineQuantity newQuantity)
, and Model#deleteMedicine(MedicineName medicine)
respectively.
Given below is an example usage scenario and how the MedicineInventory
behaves at each step.
Step 1. The user launches the ClinicIO application. The empty MedicineInventory
will be initialized at the same time
as the ClinicIO
.
Step 2. The user executes addmed mn/Paracetamol mt/tablet ed/2 ld/8 mp/0.05 mq/1000
command to add a new Medicine
to
the MedicineInventory
. The addmed
command creates a new Medicine
newMedicine
with the fields specified by the
command and calls Model#addMedicine(Paracetamol)
. This adds a new entry into MedicineInventory
.
Step 3. The user execute listmed
command to view the list of all Medicine`s in the `MedicineInventory
.
Step 4. The user executes increasemed 1 mq/500
command to increase the current MedicineQuantity
of the Medicine
at
index 1
in the Medicine List
by 500
units. The increasemed
command first checks if the MedicineInventory
contains a Medicine
at index 1
. Next, the increasemed
command adds 500
to the current MedicineQuantity
, and
calls Model#updateMedicine(Paracetamol, increasedQuantity)
. This updates the MedicineQuantity
data attribute of the
Medicine
with MedicineName
Paracetamol
in the MedicineInventory
with the latest quantity increasedQuantity
.
In the example outlined in Step 4, if the Model#hasMedicine(Paracetamol) returns false, the MedicineInventory does
not contain a Medicine with MedicineName Paracetamol , and will not call
Model#updateMedicine(Paracetamol, increasedQuantity) . This prevents the user from adding extra quantity to a
Medicine that does not exist in the MedicineInventory .
|
Step 5. The user executes decreasemed 1 q/20
command to decrease the current MedicineQuantity
of the Medicine
at
index 1
in the Medicine List
by 20
units. The decreasemed
command first checks if the MedicineInventory
contains a Medicine
at index 1
. Next, the decreasemed
command subtracts 20
from the current MedicineQuantity
,
and calls Model#updateMedicine(Paracetamol, decreasedQuantity)
. This updates the MedicineQuantity
data attribute of
the Medicine
with MedicineName
Paracetamol
in the MedicineInventory
with the latest quantity decreasedQuantity
.
In the example outlined in Step 5, if the current value of the MedicineQuantity of the Medicine with MedicineName
Paracetamol is less than 20 , the MedicineInventory does not contain enough quantity of Medicine with
MedicineName Paracetamol to prescribe to the Patient , and will not call Model#updateMedicine(Paracetamol,
decreasedQuantity) . This prevents the user from getting a negative value for MedicineQuantity of a Medicine
in the MedicineInventory .
|
Step 6. The user executes deletemed 1
command to delete the Medicine
at index 1
in the Medicine List
. The
deletemed
command first checks if the MedicineInventory
contains a Medicine
at index 1
. Next, it calls
Model#deleteMedicine(Paracetamol)
to delete the Medicine
with MedicineName
Paracetamol
from the
MedicineInventory
. Now the MedicineInventory
is empty.
In the example outlined in Step 6, if the Model#hasMedicine(Paracetamol) returns false, the MedicineInventory does
not contain a Medicine with MedicineName Paracetamol , and will not call Model#deleteMedicine(Paracetamol,
increasedQuantity) . This prevents the user from deleting a Medicine that does not exist in the MedicineInventory .
|
Design Considerations
Aspect: How to implement different protocols for Medicine
with different MedicineType
when managing the MedicineInventory
-
Alternative 1 (current choice): Use one
MedicineInventory
and implement different protocols for eachMedicineType
.-
Pros: Uses less memory.
-
Cons: Complicates implementation.
-
-
Alternative 2: Use different
MedicineInventory
for eachMedicineType
.-
Pros: Uses more memory.
-
Cons: Eases implementation of logic for commands.
-
Aspect: How to ensure that the MedicineInventory
has sufficient MedicineQuantity
of each Medicine
at all times
-
Alternative 1: Design a predictive algorithm using data from
Analytics
.-
Pros: Ensures that
MedicineInventory
will not have too large a surplus of un-prescribedMedicine
. -
Cons: Complicates implementation of logic and incorporation of data from
Analytics
.
-
-
Alternative 2 (current choice): Maintain a fixed amount of
MedicineQuantity
for eachMedicine
in theMedicineInventory
.-
Pros: Enables logic implementation to be easier and more straightforward.
-
Cons: Introduces the risk of ending up with too large a surplus of un-prescribed
Medicine
.
-